A Symbol That Defines a Math Calculation Is Python: Explain!
In Python, a variety of symbols are used to define and perform mathematical calculations within the programming language.
These include symbols for basic arithmetic like addition (+), subtraction (-), multiplication (*), and division (/), as well as symbols for more complex operations such as exponentiation (**), modulus (%), and floor division (//).
By using these symbols, programmers can execute a wide range of mathematical operations, making Python a powerful tool for numerical computing.
Python uses specific symbols to represent different mathematical operations:
Python’s mathematical symbols enable concise and intuitive numerical computations, streamlining coding tasks.
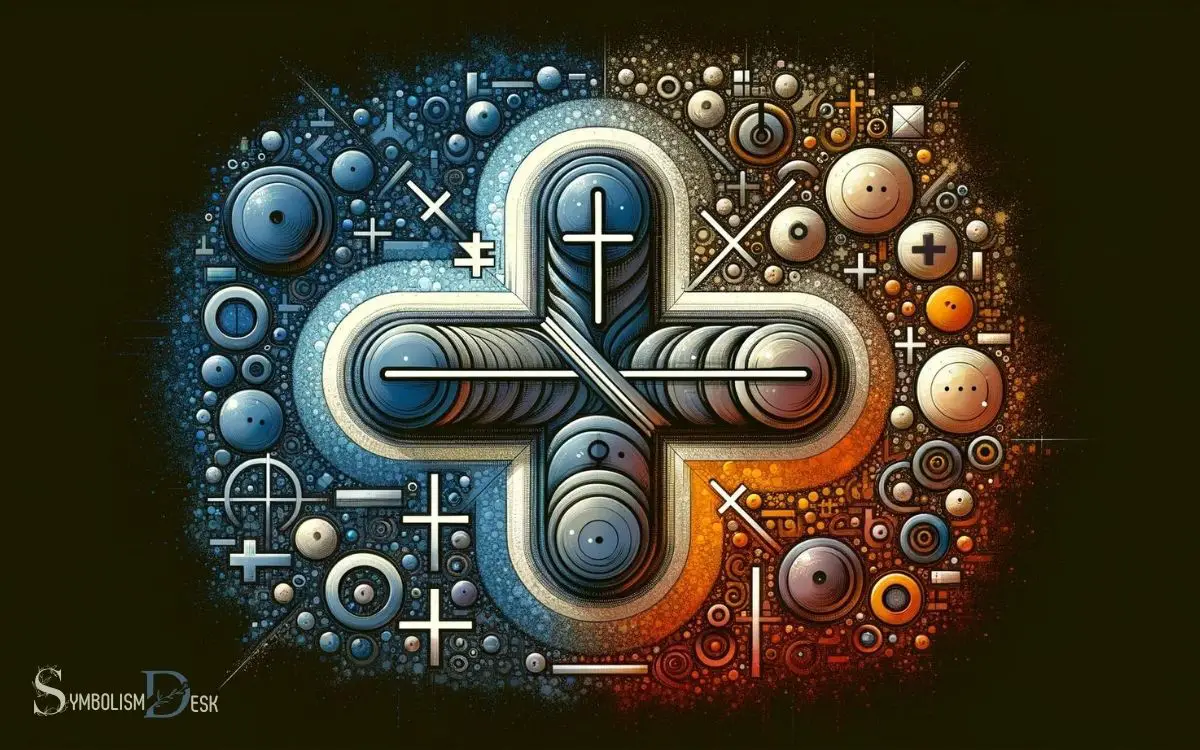
Key Takeaway
Python Mathematical Symbols and Their Uses
Symbol | Operation | Example Use | Result |
---|---|---|---|
+ | Addition | 10 + 5 | 15 |
– | Subtraction | 10 – 5 | 5 |
* | Multiplication | 10 * 5 | 50 |
/ | Division | 10 / 5 | 2.0 |
// | Floor Division | 10 // 5 | 2 |
% | Modulus | 10 % 5 | 0 |
** | Exponentiation | 10 ** 5 | 100000 |
The Origin of Python Symbol
The origin of the Python symbol can be traced back to its introduction in the programming language by Guido van Rossum in the late 1980s.
As a tribute to the British comedy group Monty Python, Rossum chose the name “Python” for its simplicity and humor, reflecting the core values of the language.
The symbol itself, a snake, was inspired by the same comedic influence and was adopted as the official logo for Python.
The choice of the snake symbol also alludes to the flexibility and agility of the language, emphasizing its ease of use and adaptability for various programming tasks.
Understanding the origin of the Python symbol provides insight into the playful and innovative spirit that underpins the language’s development.
This sets the stage for exploring its practical applications, including mathematical operations in Python.
Mathematical Operations in Python
The mathematical operations in Python encompass a wide range of functionalities, from basic arithmetic to advanced math functions and numeric data manipulation.
Understanding the fundamental principles of basic arithmetic forms the foundation for more complex mathematical operations in Python.
Additionally, Python provides powerful tools for manipulating numeric data, allowing for efficient and effective mathematical calculations and analysis.
Basic Arithmetic in Python
Basic arithmetic operations in Python encompass addition, subtraction, multiplication, and division. These operations are fundamental in mathematical calculations and are straightforward to implement in Python.
The addition operator (+) is used to add two or more numbers, while the subtraction operator (-) subtracts the second operand from the first.
The multiplication operator (*) performs multiplication on the operands, and the division operator (/) divides the first operand by the second.
Additionally, the modulo operator (%) returns the remainder of the division. Python also supports exponentiation with the double asterisk operator (**), which raises the first operand to the power of the second.
Understanding these basic arithmetic operations forms the foundation for more complex mathematical manipulations in Python, making it a versatile and powerful tool for mathematical calculations.
Advanced Math Functions
Python provides a comprehensive set of advanced math functions to facilitate complex mathematical operations with precision and efficiency.
These functions are part of the Python math module and cover a wide range of mathematical operations, including trigonometric, logarithmic, exponential, and hyperbolic functions, among others.
Below is a table summarizing some of the key advanced math functions available in Python:
Function | Description |
---|---|
math.sin(x) | Returns the sine of x |
math.cos(x) | Returns the cosine of x |
math.tan(x) | Returns the tangent of x |
math.log(x, base) | Returns the logarithm of x with base |
math.exp(x) | Returns e raised to the power of x |
These functions are invaluable for scientific and engineering applications, enabling users to perform intricate calculations with ease and accuracy.
Numeric Data Manipulation
Numeric data manipulation in Python involves performing various mathematical operations with precision and efficiency, building on the advanced math functions discussed in the previous subtopic.
When working with numeric data in Python, it’s essential to understand and utilize the following:
Basic Arithmetic Operations:
- Addition, subtraction, multiplication, and division are fundamental operations for manipulating numeric data.
Advanced Mathematical Functions:
Python provides libraries like NumPy and SciPy for advanced mathematical functions such as trigonometric functions, exponential and logarithmic functions, and more.
These libraries offer extensive capabilities for complex numerical computations, making them indispensable for scientific and engineering applications. Understanding these libraries is crucial for efficient numeric data manipulation in Python.
Python Symbol for Logical Comparisons
Python provides a range of logical comparison operators that are integral to decision-making in programming. Understanding these operators is crucial for effective programming and data analysis.
Python’s Logical Comparison Operators
The logical comparison operators in Python provide a concise and powerful way to perform comparisons between values. They are essential for decision-making and flow control in programs.
Here are two essential subtopics to understand Python’s logical comparison operators:
- Comparison Operators:
- These include ‘==’, ‘!=’, ‘<’, ‘>’, ‘<=’, and ‘>=’. They are used to compare values and return a Boolean result.
- Logical Operators:
- These include ‘and’, ‘or’, and ‘not’. They are used to combine conditional statements.
Understanding these operators is crucial for writing efficient and effective code, as they allow for complex decision-making and filtering of data.
This sets the stage for the subsequent section about the importance of logical comparisons in Python.
Importance of Logical Comparisons
Logical comparisons play a crucial role in Python for evaluating conditions and making decisions within programs.
Python provides several logical comparison operators to compare values. These operators return a Boolean value of True or False based on the comparison.
The following table summarizes the logical comparison operators in Python:
Operator | Description |
---|---|
== | Equal to |
!= | Not equal to |
< | Less than |
> | Greater than |
These operators are used to compare values and variables, allowing the program to make decisions based on the comparison results.
Understanding and using these logical comparison operators are fundamental for writing effective and efficient Python programs.
Examples of Logical Comparisons
An understanding of logical comparison operators is essential for writing effective and efficient Python programs, and in this section, we will explore three fundamental examples.
Example 1:
- Comparison: Greater Than
- Python Symbol: >
- Explanation: Compares if one value is greater than another.
Example 2:
- Comparison: Equal To
- Python Symbol: ==
- Explanation: Checks if two values are equal.
Example 3:
- Comparison: Not Equal To
- Python Symbol: !=
- Explanation: Determines if two values are not equal.
Understanding these examples will enable programmers to effectively utilize logical comparison operators in Python, leading to the development of robust and efficient code.
Python Symbol in Expressing Equations
Using a gerund noun, expressing equations with Python involves understanding the syntax and operators used for mathematical calculations.
Python provides a wide range of symbols and operators that enable the expression of complex equations in a concise and readable manner.
The following table illustrates some of the commonly used symbols and operators in Python for expressing equations:
Symbol/Operator | Description | Example |
---|---|---|
+ | Addition | x + y |
– | Subtraction | x – y |
* | Multiplication | x * y |
/ | Division | x / y |
% | Modulus | x % y |
** | Exponentiation | x ** y |
Understanding and utilizing these symbols and operators is fundamental in expressing mathematical equations effectively in Python.
Significance of Python Symbol in Programming
The utilization of Python symbols and operators is integral to programming, enabling the implementation of mathematical calculations and algorithms with precision and efficiency.
The significance of Python symbols in programming is profound and can be understood in the following ways:
- Expressiveness: Python symbols allow for concise and readable code, making it easier to express complex mathematical operations.
- For example, the use of the ‘+’ symbol for addition and the ‘-’ symbol for subtraction simplifies the representation of mathematical equations.
- Versatility: Python symbols provide a wide range of operations, including arithmetic, comparison, and logical operations, making it a versatile language for various programming tasks.
- This versatility allows programmers to manipulate data and control program flow effectively.
Python Symbol’s Role in Digital Mathematics
The utilization of Python symbols in programming encompasses a multitude of applications in digital mathematics, reflecting its fundamental role in numerical computations and algorithmic implementations.
Python’s symbols, such as +, -, *, and /, are crucial in performing basic arithmetic operations as well as more complex mathematical calculations.
In digital mathematics, Python symbols play a pivotal role in expressing mathematical equations and algorithms, enabling the manipulation and analysis of numerical data.
The table below illustrates the basic arithmetic operations using Python symbols, showcasing their significance in digital mathematics. The Python symbols demonstrated in the table simplify complex calculations and allow for efficient problem-solving in programming. Each operation can be applied to different types of numbers in math, such as integers, floating-point numbers, and even complex numbers, broadening their utility. By understanding these operations, users can manipulate data effectively and perform a wide range of computational tasks.
Operation | Symbol |
---|---|
Addition | + |
Subtraction | – |
Multiplication | * |
Division | / |
Conclusion
The Python symbol plays a crucial role in mathematical calculations and logical comparisons in programming. With its origins in the mathematical field, Python symbol has become a fundamental element in expressing equations and carrying out digital mathematics.
It is interesting to note that Python is currently one of the most popular programming languages, with over 8.2 million users worldwide, making its symbol an essential part of the digital world.