Bad Register Name Symbol Expected Integer Register: Explain!
The ‘Bad Register Name Symbol Expected Integer Register’ error is a specific programming issue that occurs when an assembler or compiler encounters an unrecognized register name or a symbolic name where an integer register is expected.
This error can happen in assembly language programming or in specific high-level languages that allow inline assembly or direct hardware manipulation.
This error signifies that during the compilation or assembly of code, the software expected to find an integer register identifier but instead found an invalid name or symbol.
Here are some common causes and scenarios:
eaxx
instead of eax
in assembly language).Understanding register usage and syntax conventions is essential to avoid the ‘Bad Register Name Symbol Expected Integer Register’ error.
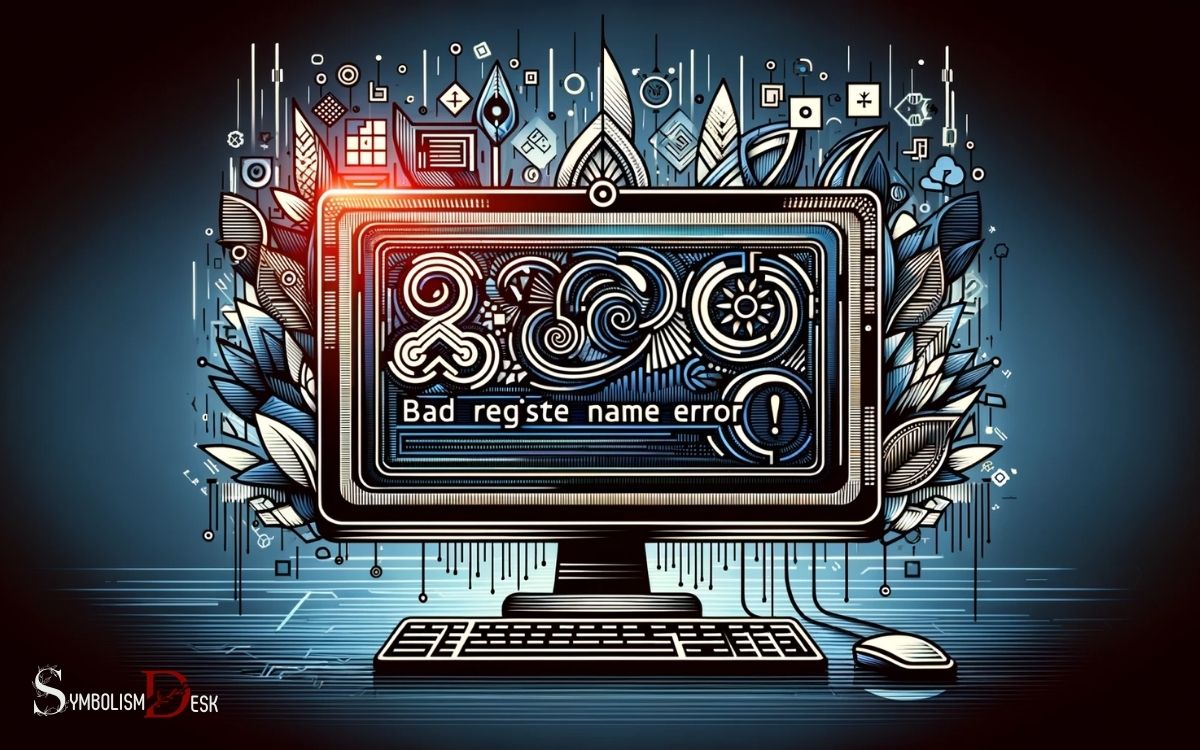
Key Takeaway
Understanding the ‘Bad Register Name Symbol Expected Integer Register’ Error
Common Causes | Explanation | Example Error Context |
---|---|---|
Typographic Error | Misspelling or incorrect register identifier | Using eaxx instead of eax |
Unsupported Register Name | Using a register name not supported by the architecture | Using r8 in a 32-bit x86 context |
Incorrect Use of Symbolic Names | Symbolic names which are not mapped to registers | Declaring myRegister without mapping |
Variable Used Instead of Register Identifier | Variables being used in place of integer register identifiers | Using intVar where eax is expected |
Understanding the Error Message
The error message ‘Bad Register Name Symbol Expected Integer Register’ indicates a requirement for an integer register instead of the expected symbol.
This error typically occurs in programming or computing environments where a specific type of register is required to perform an operation, but a symbol has been provided instead.
This discrepancy can lead to malfunctioning of the program or system, causing unexpected behavior.
Understanding the error message is crucial for troubleshooting and resolving the issue effectively.
In the subsequent section, we will delve into the causes of this error and explore the specific scenarios in which it commonly occurs, providing valuable insights for addressing and preventing such issues in the future.
Causes of the Error
When encountering the error message ‘Bad Register Name Symbol Expected Integer Register’, the causes of this issue can be attributed to various factors within the programming or computing environment.
The following factors may contribute to this error:
- Incorrect Syntax: Misuse or incorrect syntax of the register name or symbol in the code can lead to this error, causing the program to expect an integer register instead.
- Data Type Mismatch: Attempting to use a non-integer register where an integer register is expected can trigger this error, highlighting a data type mismatch within the code.
- Compiler or Assembler Limitations: Certain compilers or assemblers may have limitations or specific requirements regarding register names and symbols, which, if not adhered to, could result in this error message.
Understanding these potential causes can aid in effectively troubleshooting and resolving the ‘Bad Register Name Symbol Expected Integer Register’ error.
Common Scenarios and Examples
In programming, encountering the error ‘Bad Register Name Symbol Expected Integer Register’ can often occur in common scenarios involving register usage and symbol interpretation.
One common scenario is when attempting to access a register with an incorrect name or symbol representation, leading to the error.
For example, mistakenly using a non-integer register name or expecting a symbol where an integer register is required can trigger this error.
Another scenario involves misinterpreting the syntax for specifying integer registers, such as using incorrect punctuation or missing essential identifiers.
Additionally, attempting to perform operations that require integer registers with non-integer data can also lead to this error.
Understanding these common scenarios and examples can help programmers identify and rectify the causes of the ‘Bad Register Name Symbol Expected Integer Register’ error effectively.
Impact on Program Execution
The impact of bad register name symbol expected integer register can be severe, leading to unexpected program termination, incorrect data processing, and unpredictable program behavior.
These issues can disrupt the normal flow of program execution, leading to errors, crashes, or unintended results.
Understanding and addressing the impact of these issues is crucial for maintaining the stability and reliability of software systems.
Unexpected Program Termination
Unexpected program termination impacts program execution significantly. Such terminations can be frustrating and detrimental to the overall user experience.
The following points highlight the emotional impact of unexpected program termination:
- Frustration: Sudden program termination can lead to frustration among users, especially if they lose unsaved work or are unable to complete critical tasks.
- Anxiety: Users may experience anxiety when unexpected terminations occur, particularly if the program is essential for their work or personal needs.
- Lack of Trust: Repeated unexpected terminations can erode the trust users have in the program, leading to reluctance in using it and seeking alternative solutions.
Understanding the emotional toll of unexpected program termination emphasizes the importance of ensuring program stability and reliability.
Incorrect Data Processing
Incorrect data processing can significantly disrupt program execution, leading to unpredictable outcomes and potential system instability.
When data is processed incorrectly, it can result in erroneous calculations, memory corruption, and unexpected behavior within the program. This can lead to the program producing incorrect results or even crashing unexpectedly.
Furthermore, incorrect data processing can introduce vulnerabilities that may be exploited by malicious entities, compromising the security of the system.
It is essential for developers to diligently validate and sanitize input data, use appropriate data types, and implement error-checking mechanisms to ensure accurate data processing.
Additionally, thorough testing and debugging procedures should be employed to detect and rectify any instances of incorrect data processing, thereby promoting the stability and reliability of the program.
Unpredictable Program Behavior
During program execution, unpredictable behavior can have a significant impact on the overall performance and stability of the system. This can lead to frustration, anxiety, and decreased productivity for users and developers alike.
The uncertainty surrounding the outcome of program execution can be disheartening and can erode confidence in the reliability of the software.
Unpredictable behavior also introduces the risk of data corruption, security vulnerabilities, and system crashes, further exacerbating the negative impact on the program’s operation.
Additionally, the potential for unpredictable program behavior can create challenges in accurately assessing and addressing issues, prolonging the resolution process and hindering progress.
Understanding the causes and mitigating factors of unpredictable program behavior is crucial for maintaining the integrity and functionality of software systems.
To address these challenges, it is important to employ effective debugging and troubleshooting strategies.
Debugging and Troubleshooting Tips
One important debugging and troubleshooting tip is to thoroughly review the assembly code for any inconsistencies or errors.
This involves scrutinizing the code line by line to identify any potential issues such as incorrect register names, missing symbols, or unexpected integer register references.
Additionally, utilizing debugging tools and stepping through the code can help pinpoint the exact location of the problem. It’s also beneficial to examine any recent changes made to the code that could have introduced errors.
By carefully examining the assembly code and actively debugging the program, developers can effectively identify and address issues that may be causing the unpredictable program behavior.
Best Practices for Prevention
To prevent issues such as incorrect register names, missing symbols, or unexpected integer register references, it is essential to implement proactive strategies and best practices during the coding and debugging processes.
- Consistent Code Reviews: Regular and thorough code reviews by peers can help catch potential issues related to register names, symbols, and integer references before they cause problems in the system.
- Use of Linters and Static Code Analysis Tools: Incorporating linters and static code analysis tools into the development workflow can help identify and rectify register name symbol and integer register issues early in the coding process.
- Comprehensive Testing: Implementing comprehensive testing procedures, including unit testing, integration testing, and system testing, can help uncover and address any register-related issues before they manifest in the production environment.
Handling the Error in Different Languages
How can the error of bad register name symbol expected integer register be effectively handled in different programming languages?
In Python, you can use a try-except block to catch the error and handle it gracefully, providing a meaningful error message to the user.
Java also offers try-catch blocks for exception handling, allowing you to specify different courses of action based on the type of error.
In C++, you can use the try-catch mechanism similar to Java to handle the error and prevent program termination. JavaScript provides the try-catch statement for catching and handling exceptions.
Each of these languages offers its own syntax and best practices for error handling, allowing developers to effectively manage the bad register name symbol expected integer register error.
Advanced Techniques for Resolution
When dealing with the error of bad register name symbol expected integer register, programmers can employ advanced techniques for resolution by implementing more sophisticated error handling strategies.
To effectively address this issue, programmers can consider the following advanced techniques:
- Dynamic Error Handling: Implementing dynamic error handling techniques allows for real-time monitoring and adjustment of error handling processes, providing a more adaptable and responsive approach to resolving errors.
- Automated Error Reporting: Utilizing automated error reporting systems can streamline the process of identifying and addressing errors, enabling prompt resolution and reducing the impact of errors on system performance.
- Predictive Analysis: By employing predictive analysis tools, programmers can anticipate potential errors and proactively implement measures to mitigate their impact, enhancing system reliability and stability.
Conclusion
The error “bad register name symbol expected integer register” can cause frustration for programmers, but understanding its causes and implementing best practices for prevention can help minimize its occurrence.
Just as a skilled chef carefully selects and organizes their ingredients to create a delicious dish, a programmer must carefully manage their code to avoid errors.
By following best practices and using advanced techniques for resolution, programmers can avoid the pitfalls of this error and create successful programs.